1) Create a new Movie symbol, name it as button, and make sure the Export for ActionScript and Export in first frame options are selected.
2) Create 3 layers. Rename layers to Button Text, Button Image, and Label respectively.
3) Go to Label Layer, frame 1, change the frame label to _up. Go to frame 10, insert new keyframe, change the frame label to _over. Go to frame 20, insert new keyframe, change the frame label to _down. Go to frame 30, insert new frame.
4) Go to Button Image Layer, frame 1, draw an image for the button normal state (_up). Go to frame 10, insert blank keyframe, draw an image for the button mouse over state (_over). Go to frame 20, insert blank keyframe, draw an image for the button pressed state (_down). Go to frame 30, insert new frame.
5) Goto Button Text Layer, frame 1, add Text to the stage. Change the text to Button. Align Text properly towards to the button. Change the Text instance name to Caption. Go to frame 30, insert new frame. Below is the result:
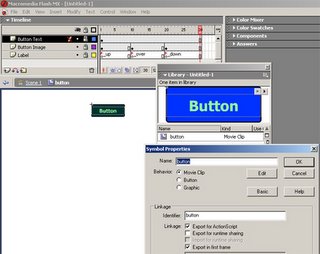
There are 10 frames between each state. But the number of frames between each state can be as low as 1. Adding more frames is just to enable the Frame Labels to be more visible (readable). To purpose to label frames to _up, _over, and _down is to enable Flash to automatically go to the correct frame for the correct button state (Flash will response to the correct state automatically if this 3 labels exists).
Following code shows how to attach Movie Clip Button to the main timeline, or to other movie clip:
// Attach movie, button is the Symbol Name attachMovie("button", "SampleButton", 1); // Set button location SampleButton._x = 10; SampleButton._y = 10; // Button text. Caption is the name given // to the Text object in the SampleButton.Caption.text = "Click Here"; // Stop the button timeline from playing. SampleButton.stop(); // Below handler is important. Flash will only // make the movie clip to be button after at least // one button event handlers has been defined SampleButton.onPress = function() { // do something here after button pressed }